Performance Tips in React.js
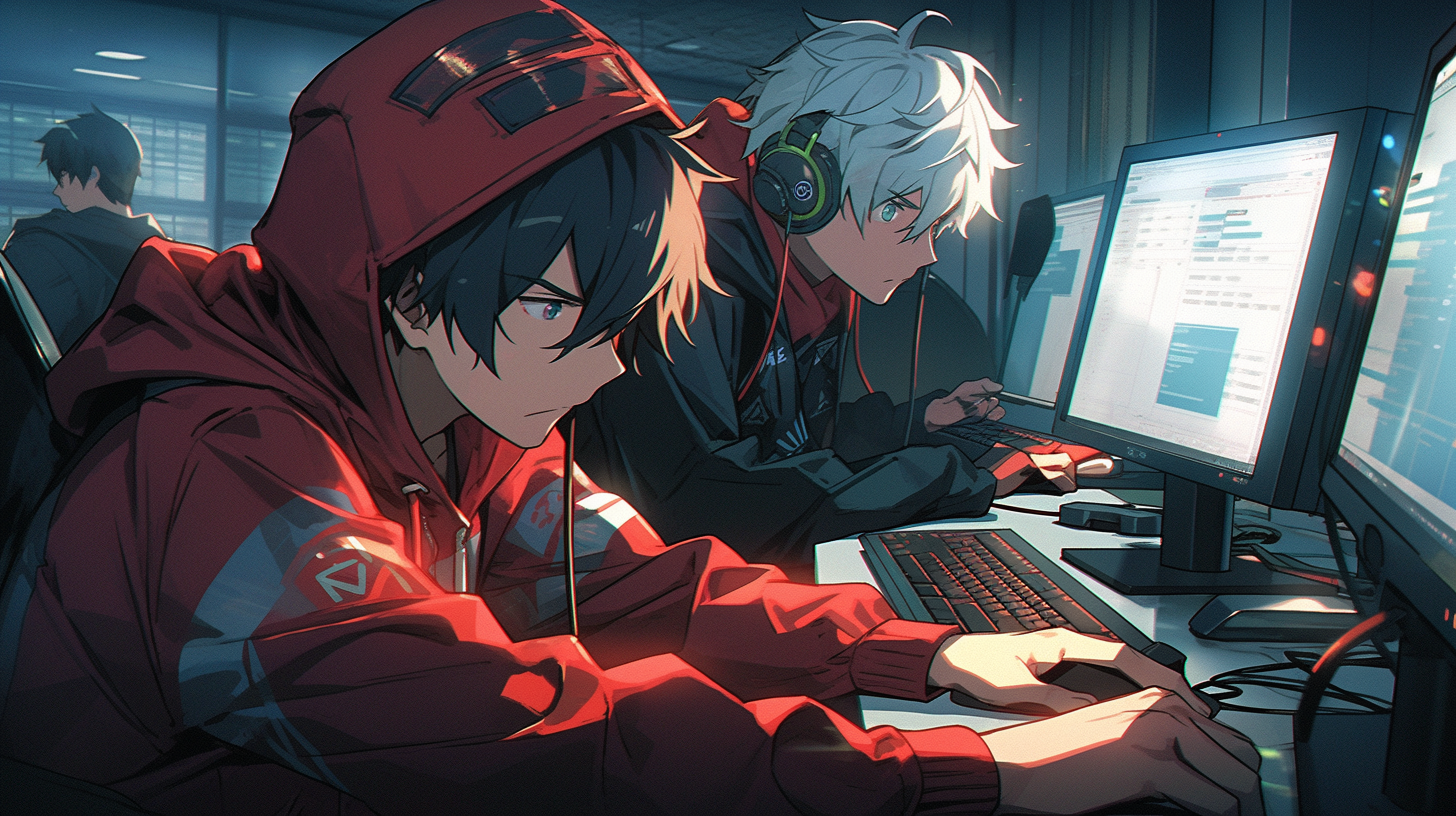
Haz clic para leer esta publicación en español.
Applying this technique can lead to a significant performance boost.
Code splitting
Implementing this technique can yield a substantial performance boost. The concept behind code splitting is to lazy load the code only when it's needed. For example, if a module in our project uses a large PDF rendering library, there's no need to render any PDFs during login, so it's much better not to load the pdfjs library. That's the essence of this technique, which is based on JavaScript standards and has syntax like this:
import('/module.js').then( module => { // do something with the module}, error => { // an error occurred while exporting the module})
We can bring this to React using the React.lazy library, which allows us to load components on-demand. Here's the trick:
const Mycomponent = React.lazy(() => import('../mymodule'))
In the JSX part of the component, we'll need to use suspense, otherwise, React will try to render our component even if it hasn't been completely loaded. React.suspense delays the rendering of our component until a condition is met and shows a fallback state (this fallback can be text, a div, or another component).
javascript
}>
This way, our component initially shows a div with the text "Loading...", and once the library is fully loaded, it displays MyComponent. We can creatively add conditionals to show certain components, like a checkbox if we want MyComponent to be loaded or whatever else; the sky's the limit.
Eager Loading
This technique allows us to load the library "hurriedly," i.e., before the user needs it. If we somehow detect that the user intends to use a module or meets the condition to load the library, then we can preload it so the user doesn't have to wait (we never want the user to wait). For example:
// declare a function that imports the library
const loadMyComponent = () => import('../mymodule')
const Mycomponent = React.lazy(loadMyComponent)
In our JSX, we can trigger our loadMyComponent function when the user hovers over a button, for example:
}>